21. November 2010
For my drawing program I wanted to revisit Rekursive functions as well as work with the sliders. My concept was to have a control field with sliders to control how many branches and flowers a generated tree should have. Once the user clicks on the "drawing board" a plant is generated with their slider input. I am still having problems with the amount of petals on the tree. But I learned alot with this excercise. Even simple ways to convert a float value to int. Here are a few pictures of the program at work.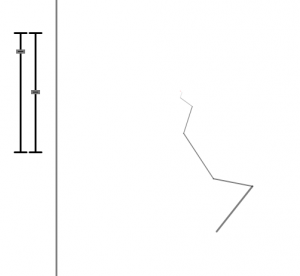
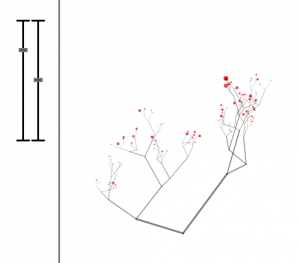
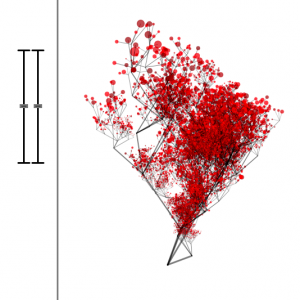
vSlider sliderR = null; vSlider sliderG = null; int x = 00; int y = 00; int w = 120; int h = 600; void setup() { size(600,600); // def. fenstergroesse randomSeed(millis()); // seed random smooth(); // aktiviere antialiasing strokeWeight(3); // linienbreite stroke(0,0,0,150); sliderR = new vSlider(50,100,20,200,.5); sliderG = new vSlider(75,100,20,200,.5); noLoop(); } void draw() { background(255); // Changed Multiplication nr on slider so that the nr is masimum 8. So 8 branches are drawn. // background(sliderR.pos() * 255,sliderG.pos() * 255); // background(sliderR.pos() * 8,sliderG.pos() * 255); // Checks the X and Y co-ordinates of the mouse to make sure that the mouse is being clicked in the correct place if ((mouseX > x) && (mouseX > x+w) && (mouseY > y) && (mouseY < y+h)) { // println("DrawingPad"); //Draws the Flower in the correct spot on the drawing board. pushMatrix(); translate(mouseX,mouseY); //translate(width *.5,height - 20); wurzel(7); popMatrix(); } else { fill (255); } rect (x, y, w, h); sliderR.draw(); sliderG.draw(); } void mousePressed() { redraw(); sliderR.mousePos(mouseX,mouseY,true); sliderG.mousePos(mouseX,mouseY,true); if (mouseEvent.getClickCount()==2) { sliderR.mouseDClick(mouseX, mouseY); sliderG.mouseDClick(mouseX, mouseY); println("<double click>"); } } void mouseDragged() { sliderR.mousePos(mouseX,mouseY,true); sliderG.mousePos(mouseX,mouseY,true); } // funktion void wurzel(int tiefe) { if(tiefe <=0) // teste ob das ende erreicht worden ist { // zeichen blueten pushStyle(); int clr = (int)random(100,255); stroke(clr,0,0,190); fill(clr,0,0,190); ellipse(0,0,50,50); popStyle(); return; } // zeichne zweige int x; int y; //int count = sliderR.pos() * 8; Cannot convert from float to int float r = sliderR.pos() * 8; int m = int(r); int count = m; // int count = (int)random(1,8); println (m); for(int i = 0; i < count;i++) { x = (int)random(-100,100); y = -(int)random(10,150); line(0,0,x,y); pushMatrix(); translate(x,y); scale(random(.3,.95)); wurzel(tiefe-1); popMatrix(); } }Here is the used Class vSlider
// Altered Slider Class : Making the Sliders Vertical class vSlider { PVector _p1; PVector _p2; int _h; int _w; float _pos; vSlider(int x,int y,int w,int h,float pos) { _p1 = new PVector(x + w * .5,y); _p2 = new PVector(x + w * .5,y + h); /* _p1 = new PVector(x,y + h *.5); _p2 = new PVector(x+w,y + h *.5); */ _w = w; _pos = pos; if(_pos > 1.0) _pos = 1.0; else if(_pos < 0.0) _pos = 0.0; } float pos() { return _pos; } boolean isHit(int x,int y) { if(y > _p1.y && y < _p2.y && x > _p1.x - 5 && x < _p1.x + 5) return true; else return false; } void draw() { pushStyle(); drawSlider(); drawKnob(); popStyle(); } void drawSlider() { stroke(0); line(_p1.x,_p1.y,_p2.x,_p2.y); line(_p1.x - _w *.5,_p1.y,_p1.x +_w * .5,_p1.y); line(_p2.x - _w *.5,_p2.y,_p2.x +_w * .5,_p2.y); } void drawKnob() { stroke(100); fill(0); PVector dir = PVector.sub(_p2,_p1); PVector pos = PVector.add( _p1 , PVector.mult(dir,_pos)); rect(pos.x-6,pos.y-3,12,4); } void mousePos(int x,int y,boolean pressed) { if(pressed) { if(isHit(x,y)) { // move knob PVector dir = PVector.sub(_p2,_p1); _pos = 1.0 / dir.mag() * (y - _p1.y); } } else { // mouse over } } void mouseDClick(int x, int y) { if(isHit(x,y)) { println(_pos); print(x); print(y); _pos = .5; } } }