2. Dezember 2010
Nun sollten wir das Ergebniss aus der Aufgabe Permutation mit Processing noch um eine Eingabe mittels Maus erweitern: Im Uhrzeiger Sinn Die Funktion "getMouseDegree" gibt eine Gradzahl an, in welchem Winkel eine Gerade vom Mittelpunkt zur Mouse zu einer vertikalen Geraden durch den Mittelpunkt steht:/* * GET MOUSE DEGREE */ float getMouseDegree() { float mouseDegree = degrees(atan2(mouseY - height*.5, mouseX - width*.5)); if (mouseDegree < 0) { // negative if(mouseDegree >= -90) { mouseDegree = (mouseDegree+90); } else { mouseDegree = (mouseDegree+450); } } else { mouseDegree = (mouseDegree+90); } return mouseDegree; }Fertiges Programm
/* * PERMUTATION * * Create a sign permutation with processing. * * @name Permutation * @author Sebastian Pape <sebastian.pape@zhdk.ch> * @version 0.9 */ /* * VARIABLES */ int numberPerRow = 7; int padding = 50; float xStep; float yStep; float count = 1; int[] permutationsList = { 0, 1, 2, 3, 4, 5, 6, 1, 2, 3, 4, 5, 6, 5, 2, 3, 4, 5, 6, 5, 4, 3, 4, 5, 6, 5, 4, 3, 4, 5, 6, 5, 4, 3, 2, 5, 6, 5, 4, 3, 2, 1, 6, 5, 4, 3, 2, 1, 0 }; /* * VARIABLES */ void setup() { size(600, 600); smooth(); xStep = (width - 2 * padding) / (float)(numberPerRow-1); yStep = (height - 2 * padding) / (float)(numberPerRow-1); } void draw() { background(255); int permutationsIndex = 0; pushMatrix(); translate(padding,padding); for(int y=0; y<numberPerRow;y++) { pushMatrix(); for(int x=0; x<numberPerRow;x++) { drawPermutationObj(permutationsList[permutationsIndex], radians(getMouseDegree())); ++permutationsIndex; translate(xStep,0.0f); } println(); popMatrix(); translate(0.0f,yStep); } popMatrix(); } void drawPermutationObj(int type, float transformation) { pushMatrix(); rotate(transformation); pushStyle(); switch(type) { case 0: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,58,58); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(-10,6,48,48); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(8,0,58,58); break; case 1: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,62,62); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(-13,1,49,49); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(4,5,62,62); break; case 2: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,64,64); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(-11,-12,41,41); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(-5,4,55,55); break; case 3: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,65,65); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(-3,-17,40,40); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(-4,-1,53,53); break; case 4: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,66,66); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(4,-17,38,38); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(-2,-4,45,45); break; case 5: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,67,67); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(15,-11,30,30); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(4,-4,39,39); break; case 6: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,68,68); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(23,0,29,29); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(8,0,38,38); break; default: break; } popStyle(); popMatrix(); } void keyPressed() { switch(key) { case ' ': save("permutation.jpg"); break; } } /* * GET MOUSE DEGREE */ float getMouseDegree() { float mouseDegree = degrees(atan2(mouseY - height*.5, mouseX - width*.5)); if (mouseDegree < 0) { // negative if(mouseDegree >= -90) { mouseDegree = (mouseDegree+90); } else { mouseDegree = (mouseDegree+450); } } else { mouseDegree = (mouseDegree+90); } return mouseDegree; }
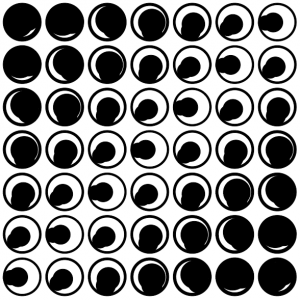
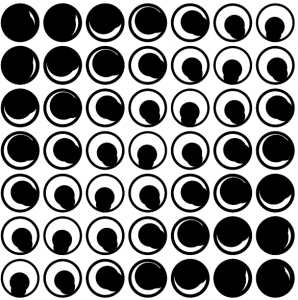
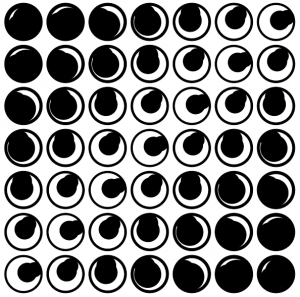
Jedes Zeichen für sich "Katze bürsten"(Max) Nun wird die Maus in Beziehung zu jedem einzelnen Zeichen gestellt und nicht nur zum Mittelpunkt des Fensters...
/* * PERMUTATION * * Create a sign permutation with processing. * * @name Permutation * @author Sebastian Pape <sebastian.pape@zhdk.ch> * @version 0.9 */ /* * VARIABLES */ int numberPerRow = 7; int padding = 50; float xStep; float yStep; float count = 1; int[] permutationsList = { 0, 1, 2, 3, 4, 5, 6, 1, 2, 3, 4, 5, 6, 5, 2, 3, 4, 5, 6, 5, 4, 3, 4, 5, 6, 5, 4, 3, 4, 5, 6, 5, 4, 3, 2, 5, 6, 5, 4, 3, 2, 1, 6, 5, 4, 3, 2, 1, 0 }; /* * VARIABLES */ void setup() { size(600, 600); smooth(); xStep = (width - 2 * padding) / (float)(numberPerRow-1); yStep = (height - 2 * padding) / (float)(numberPerRow-1); } void draw() { background(255); int permutationsIndex = 0; pushMatrix(); translate(padding,padding); for(int y=0; y<numberPerRow;y++) { pushMatrix(); for(int x=0; x<numberPerRow;x++) { print(xStep +","+ yStep + "\n"); drawPermutationObj(permutationsList[permutationsIndex], radians(getMouseDegree(xStep*x + 35, yStep*y + 35))); ++permutationsIndex; translate(xStep,0.0f); } popMatrix(); translate(0.0f,yStep); } popMatrix(); } void drawPermutationObj(int type, float transformation) { pushMatrix(); rotate(transformation); pushStyle(); switch(type) { case 0: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,58,58); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(-10,6,48,48); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(8,0,58,58); break; case 1: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,62,62); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(-13,1,49,49); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(4,5,62,62); break; case 2: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,64,64); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(-11,-12,41,41); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(-5,4,55,55); break; case 3: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,65,65); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(-3,-17,40,40); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(-4,-1,53,53); break; case 4: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,66,66); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(4,-17,38,38); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(-2,-4,45,45); break; case 5: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,67,67); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(15,-11,30,30); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(4,-4,39,39); break; case 6: /* CIRCLE 1 */ stroke(0); fill(0); ellipse(0,0,75,75); /* CIRCLE 2 */ stroke(0); fill(255); ellipse(0,0,68,68); /* CIRCLE 3 */ stroke(0); fill(0); ellipse(23,0,29,29); /* CIRCLE 4 */ stroke(0); fill(0); ellipse(8,0,38,38); break; default: break; } popStyle(); popMatrix(); } void keyPressed() { switch(key) { case ' ': save("permutation.jpg"); break; } } /* * GET MOUSE DEGREE */ float getMouseDegree(float _x, float _y) { float mouseDegree = degrees(atan2(mouseY - _y, mouseX - _x)); if (mouseDegree < 0) { // negative if(mouseDegree >= -90) { mouseDegree = (mouseDegree+90); } else { mouseDegree = (mouseDegree+450); } } else { mouseDegree = (mouseDegree+90); } return mouseDegree; }
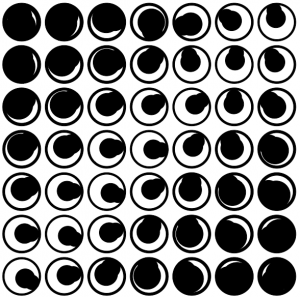
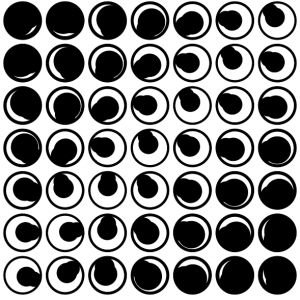
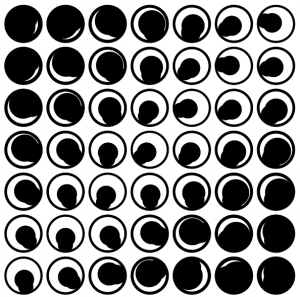