3. Januar 2011
Hier versuchte ich die Permutation in die 3 Dimension zu bringen. Der erste Hinderungsgrund war, dass ich keine Kugeln benutzen konnte. Dies war so rechnerintensiv das ich genau ein Standbild hinkriegte und ganze Kiste lahmlegte. Die Boxen haben nur einen Bruchteil von den Vektoren, leider ergibt sich da nichts schönes. Das zweite Problem war eine sauber Ausleuchtung und als letztes bekomme ich für die Interaktion von meiner Maus nur X- und Y-Werte, der Z-Wert musste simuliert werden.Funktion: Wenn sich die Maus in einen definierten Radius von einem Objekt befindet, wird dieses gezeichnet und stirbt nach einer vorgegeben Zeit (Lebenszyklus).
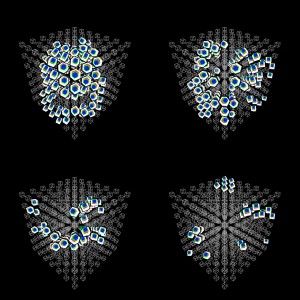
import processing.opengl.*; PVector cam; // camera position Zeichen[] voxel; int distZeichen = 20; boolean showCursor = true; int x = 7; int y = 7; int z = 7; int shift = 50; void setup() { size(500, 500, OPENGL); cam = new PVector(800,800,800); //hint(ENABLE_OPENGL_4X_SMOOTH); //hint(DISABLE_DEPTH_TEST); //smooth(); int i,j,k; int count = 0; // init zeichen voxel = new Zeichen[x*y*z]; for (i = 0; i < x; i ++ ) { for (j = 0; j < y; j ++ ) { for (k = 0; k < z; k++ ) { voxel[count] = new Zeichen(new PVector(i*shift,j*shift,k*shift),new PVector(shift,shift,shift)); count++; } } } } void draw() { if(showCursor) cursor(ARROW); else noCursor(); background(0); camera(cam.x, cam.y, cam.z, cam.x/2, cam.y/2, cam.z/2, 0.0, 0.0, -1.0); scale(1.5,1.5,1.5); noStroke(); lights(); directionalLight(cam.x, cam.y, cam.z, 0, 1, 0); ambientLight(200, 200, 200); PVector mouseCenter = new PVector(mouseX, mouseY); for(int i=0; i<voxel.length; i++){ PVector voxelCoord = voxel[i].getCoord(); PVector screenPoint = new PVector(screenX(voxelCoord.x, voxelCoord.y, voxelCoord.z),screenY(voxelCoord.x, voxelCoord.y, voxelCoord.z)); if(screenPoint.dist(mouseCenter) < distZeichen){ voxel[i].setCollision(true); } voxel[i].render(); } } void keyPressed() { switch(key) { case 'c': showCursor = !showCursor; break; } }
Klasse Zeichen:
class Zeichen { int _maxLifecycle = 100; PVector _coord; PVector _dim; float _angle; boolean _collision; int _lifecyle; // constructor Zeichen(PVector coord, PVector dim) { _coord = coord; _dim = dim; _lifecyle = 0; } void render() { if (_collision) { _lifecyle++; } if (_lifecyle > _maxLifecycle) { _lifecyle = 0; _collision = false; } noStroke(); pushMatrix(); translate(_coord.x+_dim.x/2,_coord.y+_dim.y/2,_coord.z+_dim.z/2); drawZeichen(); popMatrix(); } void drawZeichen() { pushMatrix(); if(_collision){ _angle = atan2(mouseY-_coord.y, mouseX-_coord.x); rotateX(_angle); rotateY(_angle); rotateZ(_angle); fill(0,150); translate(0,0,0); box(24); fill(255,250); translate(0,2.5* cos(2*_angle),0); box(20); fill(255, 0, 0, 150); translate(-3*cos(2*_angle), 3* sin(2*_angle), 0); box(13); fill(0, 255, 0,150); translate(3* cos(2*_angle), 3* sin(2*_angle), 0); box(13); fill(0,0,255,150); translate(-3* sin(2*_angle),0, 0); box(10); fill(255,150); translate(3* sin(2*_angle),0, 0); } else { stroke(200,100); noFill(); //point(0,0,0); box(15); } popMatrix(); } PVector getCoord() { return _coord; } void setCollision(boolean state) { _collision = state; } }