Dezember 21, 2011
Der Prototyp In dieser Woche ging es wirklich heiss zu und her. Wir erarbeiteten in dieser Woche den endgültigen Prototyp unser "El Toro 3000". Hierzu legte sich Nando unser Industrial Designer im Team mächtig ins Zeug und überraschte uns mit seiner Blechbiegekunst.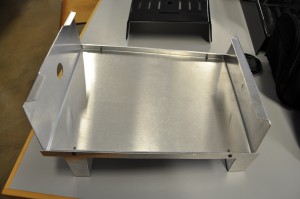
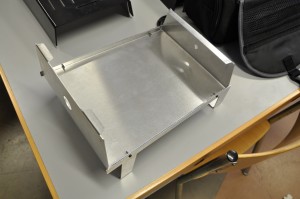
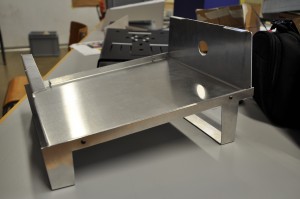
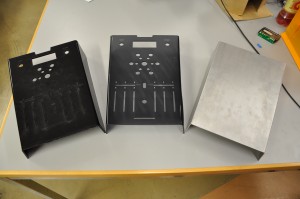
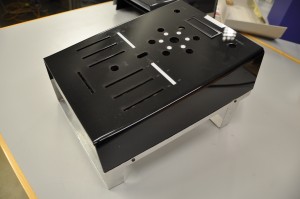
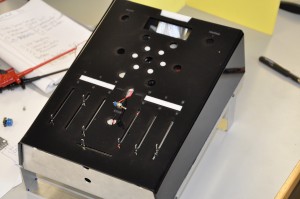
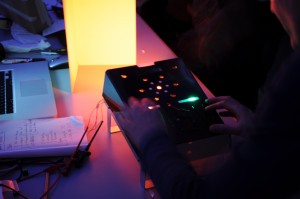
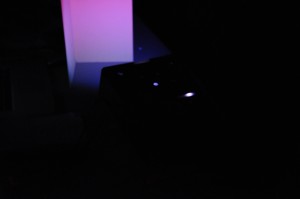
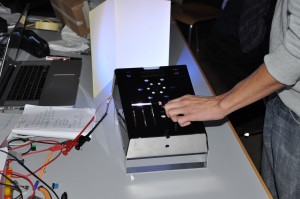
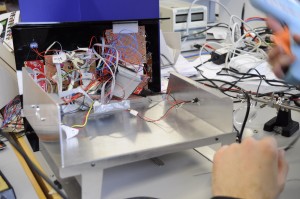
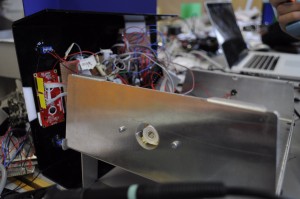
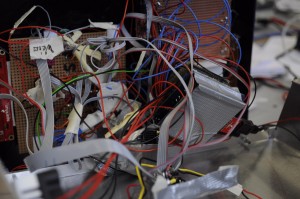
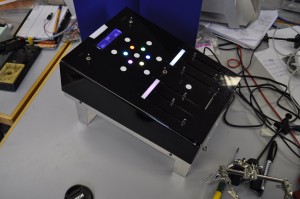
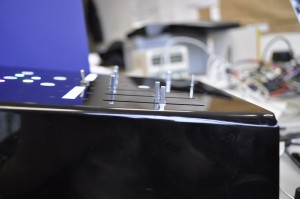
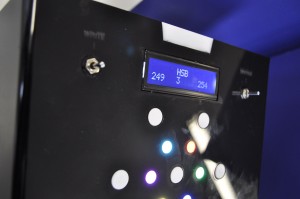
Arduino Code
// Code for the HPRGB board driving a 8.5 Watt Dealextreme LED // Input Voltage: 12V // Current per LED: 350mA // Board Frequency: 600 KHz // Vf R: 6,39 // Vf G: 9,38 // Vf B: 9,22 // AIN 4 + 5 are used for I2C communication // Libraries --------------------------------------------------------- #include <Wire.h> #include <HPRGB2.h> #include <NewSoftSerial.h> // Instance of HPRGB Object ------------------------------------------ HPRGB ledShield; // default mcp4728 id(0) and default PCA9685 id(0) // Pin definitions --------------------------------------------------- // define the pins for buttons and switches #define RGBPIN 4 #define BUTWHITE 12 #define BUTKOMP A0 // slider multiplexer #define S1 5 #define S2 6 #define S3 7 #define mp1 A3 // Common Output Analog(Z) // button multiplexer #define S4 9 #define S5 10 #define S6 11 #define mp2 A2 // Common Output Analog(Z) // define small rgb led pins #define SDI 2 #define CKI 3 #define STRIP_LENGTH 8 //LEDs on this strip // LCD Display #define txPin 8 // const const int sliderAnzahl = 7; const int buttonAnzahl = 8; const int mpSelection[][3] = { {0,0,0}, {1,0,0}, {0,1,0}, {1,1,0}, {0,0,1}, {1,0,1}, {0,1,1}, {1,1,1} }; // Variables --------------------------------------------------------- // rgb switch boolean rgbState = HIGH; // white switch boolean whiteState = HIGH; // complementar switch boolean kompState = HIGH; // sliders int sliderValue[] = {0,0,0,0,0,0,0}; // buttons boolean buttonValue[] = {false,false,false,false,false,false,false,false}; // memory mode boolean memoryMode = false; int playCounter = 2; // led out int ledOut1 = 0; int ledOut2 = 0; int ledOut3 = 0; // led backup int _ledOut1 = 0; int _ledOut2 = 0; int _ledOut3 = 0; // led complementar int ledOutKom1 = 0; int ledOutKom2 = 0; int ledOutKom3 = 0; // small LED byte rgbArray[3]; // array to store rgb values converted from hsb to rgb long strip_colors[] = {0,0,0xFF0000,0xFFFF00,0x00FF00,0x00FFFF,0x0000FF,0xFF00FF}; // array holding colors for all leds in strip long _strip_colors[] = {0,0,0,0,0,0,0,0}; // LCD Display NewSoftSerial lcd(0,txPin); // Setup ------------------------------------------------------------- void setup() { Serial.begin(9600); // Setup Serial Port // slider mp pinMode(S1, OUTPUT); // Analog Multiplexer pinMode(S2, OUTPUT); pinMode(S3, OUTPUT); // button mp pinMode(S4, OUTPUT); // Analog Multiplexer pinMode(S5, OUTPUT); pinMode(S6, OUTPUT); // RGB ledShield.begin(); ledShield.setCurrent(350,350,350); // set maximum current for channel 1-3 (mA) ledShield.setFreq(600 ); // operation frequency of the LED driver (KHz) ledShield.eepromWrite(); // write current settings to EEPROM delay(100); // wait for EEPROM writing // RGB / HSB switch pinMode(RGBPIN, INPUT); digitalWrite(RGBPIN, HIGH); // button white pinMode(BUTWHITE, INPUT); digitalWrite(BUTWHITE, HIGH); // button complementar pinMode(BUTKOMP, INPUT); // digitalWrite(BUTKOMP, HIGH); // 10k Ohm on 5V // small LED pins pinMode(SDI, OUTPUT); pinMode(CKI, OUTPUT); // init LCD lcd.begin(9600); } // Loop ------------------------------------------------------------- void loop() { // read switch mode rgbState = digitalRead(RGBPIN); whiteState = digitalRead(BUTWHITE); kompState = digitalRead(BUTKOMP); // read color for (int i = 0; i < sliderAnzahl; i++) { digitalWrite(S1, mpSelection[i][0]); digitalWrite(S2, mpSelection[i][1]); digitalWrite(S3, mpSelection[i][2]); sliderValue[i] = map(analogRead(mp1),0,1023,0,255); // 10bit -> 8bit } // map fade color ledOut1 = map(sliderValue[6],0,255,sliderValue[0],sliderValue[3]); ledOut2 = map(sliderValue[6],0,255,sliderValue[1],sliderValue[4]); ledOut3 = map(sliderValue[6],0,255,sliderValue[2],sliderValue[5]); // complementar color if(rgbState == HIGH){ ledOutKom1 = 255 - ledOut1; ledOutKom2 = 255 - ledOut2; ledOutKom3 = 255 - ledOut3; } else { ledOutKom1 = ledOut1 + 128; if(ledOutKom1 >= 255) ledOutKom1-= 255; ledOutKom2 = ledOut2; ledOutKom3 = ledOut3; } ledOut1 = 0.2*ledOut1 + 0.8*_ledOut1; ledOut2 = 0.2*ledOut2 + 0.8*_ledOut2; ledOut3 = 0.2*ledOut3 + 0.8*_ledOut3; // clear buttons & memorymode if(((ledOut1-_ledOut1) > 5 || (ledOut1-_ledOut1) < -5) || ((ledOut2-_ledOut2) > 5 || (ledOut2-_ledOut2) < -5) || ((ledOut3-_ledOut3) > 5 || (ledOut3-_ledOut3) < -5) ||) { memoryMode = false; for(int i=0;i < buttonAnzahl; i++ ) { buttonValue[i] = false; } } // color channel backup for smoothing _ledOut1 = ledOut1; _ledOut2 = ledOut2; _ledOut3 = ledOut3; if(kompState == LOW) { if(rgbState == HIGH){ writeToLCD(1,(int)ledOutKom1,(int)ledOutKom2,(int)ledOutKom3); ledShield.goToRGB(ledOutKom1,ledOutKom2,ledOutKom3); } else { writeToLCD(1,(int)ledOutKom1,(int)ledOutKom2,(int)ledOutKom3); ledShield.goToRGB(ledOutKom1,ledOutKom2,ledOutKom3); } } else if(whiteState == LOW) { writeToLCD(1,(int)250,(int)250,(int)250); ledShield.goToRGB(250, 250, 250); } else { for (int i = 0; i < buttonAnzahl; i++) { digitalWrite(S4, mpSelection[i][0]); digitalWrite(S5, mpSelection[i][1]); digitalWrite(S6, mpSelection[i][2]); if(analogRead(mp2) for(int i=0;i < buttonAnzahl-1; i++ ) { buttonValue[i] = false; } buttonValue[i] = true; memoryMode = true; } } if(memoryMode) { // save button 1 if(buttonValue[0] && buttonValue[7]) { strip_colors[2] = (long)ledOut1 << 16 | (long)ledOut2 << 8 | (long)ledOut3; buttonValue[7] = false; buttonValue[0] = false; memoryMode = false; } else if(buttonValue[0]) { ledOut1 = strip_colors[2] >> 16 & 0xff; ledOut2 = strip_colors[2] >> 8 & 0xff; ledOut3 = strip_colors[2] & 0xff; } // save button 2 if(buttonValue[1] && buttonValue[7]) { strip_colors[3] = (long)ledOut1 << 16 | (long)ledOut2 << 8 | (long)ledOut3; buttonValue[7] = false; buttonValue[1] = false; memoryMode = false; } else if(buttonValue[1]) { ledOut1 = strip_colors[3] >> 16 & 0xff; ledOut2 = strip_colors[3] >> 8 & 0xff; ledOut3 = strip_colors[3] & 0xff; } // save button 3 if(buttonValue[2] && buttonValue[7]) { strip_colors[4] = (long)ledOut1 << 16 | (long)ledOut2 << 8 | (long)ledOut3; buttonValue[7] = false; buttonValue[2] = false; memoryMode = false; } else if(buttonValue[2]) { ledOut1 = strip_colors[4] >> 16 & 0xff; ledOut2 = strip_colors[4] >> 8 & 0xff; ledOut3 = strip_colors[4] & 0xff; } // save button 4 if(buttonValue[3] && buttonValue[7]) { strip_colors[5] = (long)ledOut1 << 16 | (long)ledOut2 << 8 | (long)ledOut3; buttonValue[7] = false; buttonValue[3] = false; memoryMode = false; } else if(buttonValue[3]) { ledOut1 = strip_colors[5] >> 16 & 0xff; ledOut2 = strip_colors[5] >> 8 & 0xff; ledOut3 = strip_colors[5] & 0xff; } // save button 5 if(buttonValue[4] && buttonValue[7]) { strip_colors[6] = (long)ledOut1 << 16 | (long)ledOut2 << 8 | (long)ledOut3; buttonValue[7] = false; buttonValue[4] = false; memoryMode = false; } else if(buttonValue[4]) { ledOut1 = strip_colors[6] >> 16 & 0xff; ledOut2 = strip_colors[6] >> 8 & 0xff; ledOut3 = strip_colors[6] & 0xff; } // save button 6 if(buttonValue[5] && buttonValue[7]) { strip_colors[7] = (long)ledOut1 << 16 | (long)ledOut2 << 8 | (long)ledOut3; buttonValue[7] = false; buttonValue[5] = false; memoryMode = false; } else if(buttonValue[5]) { ledOut1 = strip_colors[7] >> 16 & 0xff; ledOut2 = strip_colors[7] >> 8 & 0xff; ledOut3 = strip_colors[7] & 0xff; } // play if(buttonValue[6]) { for(int i=0;i < buttonAnzahl; i++ ) {buttonValue[i] = false;} buttonValue[6] = true; for (int i = 0; i < STRIP_LENGTH; i++) { _strip_colors[i] = strip_colors[i]; if(playCounter != i){ strip_colors[i] = 0; } } ledOut1 = strip_colors[playCounter] >> 16 & 0xff; ledOut2 = strip_colors[playCounter] >> 8 & 0xff; ledOut3 = strip_colors[playCounter] & 0xff; writeToLCD(1,(int)ledOut1,(int)ledOut2,(int)ledOut3); ledShield.goToRGB(ledOut1, ledOut2, ledOut3); post_frame(); delay(1000); playCounter++; if(playCounter >= STRIP_LENGTH) {playCounter = 2; } for (int i = 0; i < STRIP_LENGTH; i++) { strip_colors[i] = _strip_colors[i]; } } // save if(buttonValue[7]) { for (int i = 2; i < STRIP_LENGTH; i++) { _strip_colors[i] = strip_colors[i]; strip_colors[i] = 0; } //delay(10); post_frame(); for (int i = 0; i < STRIP_LENGTH; i++) { strip_colors[i] = _strip_colors[i]; } post_frame(); } } else { // live mode // rgb / hsb if (rgbState == HIGH){ writeToLCD(1,(int)ledOut1,(int)ledOut2,(int)ledOut3); // we are in rgb mode ledShield.goToRGB(ledOut1, ledOut2, ledOut3); //small LED strip_colors[0] = (long)sliderValue[0] << 16 | (long)sliderValue[1] << 8 | (long)sliderValue[2]; // LEFT bit shifted to one r g b value strip_colors[1] = (long)sliderValue[3] << 16 | (long)sliderValue[4] << 8 | (long)sliderValue[5]; // RIGHT bit shifted to one r g b value post_frame(); //Push the current color array to the strip }else { writeToLCD(2,(int)ledOut1,(int)ledOut2,(int)ledOut3); // we are in hsb mode ledShield.goToHSB(ledOut1, ledOut2, ledOut3); //small LED // left slider convertHSBtoRGB((byte)sliderValue[0], (byte)sliderValue[1], (byte)sliderValue[2],rgbArray); strip_colors[0] = (long)rgbArray[0] << 16 | (long)rgbArray[1] << 8 | (long)rgbArray[2]; // right slider convertHSBtoRGB((byte)sliderValue[3], (byte)sliderValue[4], (byte)sliderValue[5],rgbArray); strip_colors[1] = (long)rgbArray[0] << 16 | (long)rgbArray[1] << 8 | (long)rgbArray[2]; post_frame(); } } } } //Takes the current strip color array and pushes it out void post_frame (void) { //Each LED requires 24 bits of data //MSB: R7, R6, R5..., G7, G6..., B7, B6... B0 //Once the 24 bits have been delivered, the IC immediately relays these bits to its neighbor //Pulling the clock low for 500us or more causes the IC to post the data. for(int LED_number = 0 ; LED_number < STRIP_LENGTH ; LED_number++) { long this_led_color = strip_colors[LED_number]; //24 bits of color data for(byte color_bit = 23 ; color_bit != 255 ; color_bit--) { //Feed color bit 23 first (red data MSB) digitalWrite(CKI, LOW); //Only change data when clock is low long mask = 1L << color_bit; //The 1'L' forces the 1 to start as a 32 bit number, otherwise it defaults to 16-bit. if(this_led_color & mask) digitalWrite(SDI, HIGH); else digitalWrite(SDI, LOW); digitalWrite(CKI, HIGH); //Data is latched when clock goes high } } //Pull clock low to put strip into reset/post mode digitalWrite(CKI, LOW); delayMicroseconds(500); //Wait for 500us to go into reset } void convertHSBtoRGB(byte h, byte s, byte b, byte rgbData[]){ if ( s == 0 ){ rgbData[0] = rgbData[1] = rgbData[2] = b; } else { int i = ((unsigned int)h * 6) / 256; int f = ((unsigned int)h * 6) % 256; int p = ((unsigned int)b * (255 - (unsigned int)s)) / 256; int q = ((unsigned int)b * (255 - ((unsigned int)s * f) / 256)) / 256; int t = ((unsigned int)b * (255 - ((unsigned int)s * (255 - f)) / 256)) / 256; if ( i == 0 ) { rgbData[0] = b ; rgbData[1] = t ; rgbData[2] = p; } // 0 deg (r) to 60 deg (r+g) else if ( i == 1 ) { rgbData[0] = q ; rgbData[1] = b ; rgbData[2] = p; } // 60 deg (r+g) to 120 deg (g) else if ( i == 2 ) { rgbData[0] = p ; rgbData[1] = b ; rgbData[2] = t; } // 120 deg (g) to 180 deg (g+b) else if ( i == 3 ) { rgbData[0] = p ; rgbData[1] = q ; rgbData[2] = b; } // 180 deg (g+b) to 240 deg (b) else if ( i == 4 ) { rgbData[0] = t ; rgbData[1] = p ; rgbData[2] = b; } // 240 deg (b) to 300 deg (b+r) else { rgbData[0] = b ; rgbData[1] = p ; rgbData[2] = q; } // 300 deg (b+r) to 0 deg (r) } } // LCD void writeToLCD(int _mode, int _channel1, int _channel2, int _channel3) { lcd.print(_mode); lcd.print(","); lcd.print(_channel1); lcd.print(","); lcd.print(_channel2); lcd.print(","); lcd.print(_channel3); lcd.println(); }