Februar 26, 2012
Das folgende Processing Programm errechnet den am meisten vorkommenden Farbton im Such-Rechteck. Das Rechteck kann mit den den Pfeiltasten vergrössert und verkleiner werden.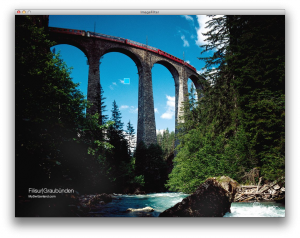
// Setings int colors = 4; int boxWidth = 20; // Objects PImage img; HashMap colorList; ArrayList mainColors; void setup() { // Setup colorMode(HSB,255); img = loadImage("image.jpg"); size(img.width,img.height); } void draw() { // Draw Image image(img,0,0); // Load Pixels loadPixels(); // Init Objects colorList = new HashMap(); mainColors = new ArrayList(); // Analyze for(int row=limit(mouseY-(boxWidth/2),0,img.height); row < limit(mouseY+(boxWidth/2),0,img.height); row++) { for(int col=limit(mouseX-(boxWidth/2),0,img.width); col < limit(mouseX+(boxWidth/2),0,img.width); col++) { // Get Info int i = (row*img.width)+col; color c = pixels[i]; int hu = int(hue(c)); // Check if(colorList.containsKey(hu)) { Increment inc = (Increment)colorList.get(hu); inc.plus(); } else { colorList.put(hu,new Increment()); } } } // Get Colors while(mainColors.size() < colors) { Iterator<Map.Entry> iterator = colorList.entrySet().iterator(); int lastCount = 0; int bigestHue = 0; while(iterator.hasNext()) { Map.Entry item = iterator.next(); int hu = (Integer)item.getKey(); int co = ((Increment)item.getValue()).get(); if(co > lastCount) { bigestHue = hu; } } mainColors.add(bigestHue); colorList.remove(bigestHue); } // Update Pixels updatePixels(); // Draw Rect rectMode(CENTER); fill(color((Integer)mainColors.get(0),255,255)); stroke(255); rect(mouseX,mouseY,boxWidth,boxWidth); } int limit(int value, int min, int max) { if(value >= min && value <= max) { return value; } else if (value > max) { return max; } else if(value < min) { return min; } return 0; } void keyPressed() { switch(keyCode) { case 40: { boxWidth -= 5; break; } case 38: { boxWidth += 5; break; } } } class Increment { int value; Increment() { this.value = 1; } void plus() { this.value++; } int get() { return this.value; } }