Februar 29, 2012
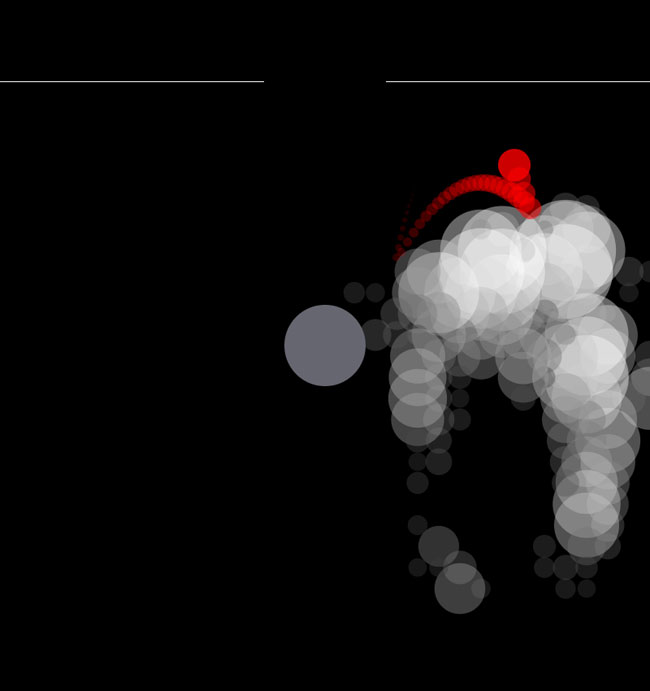
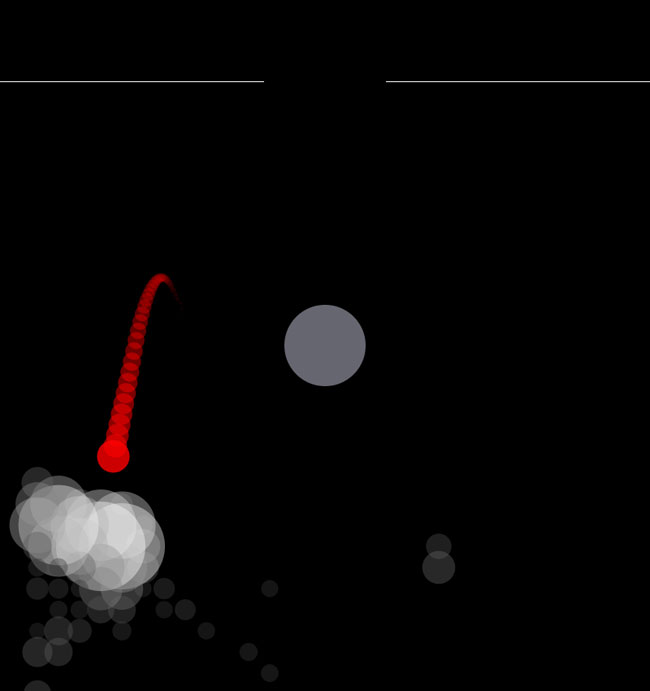
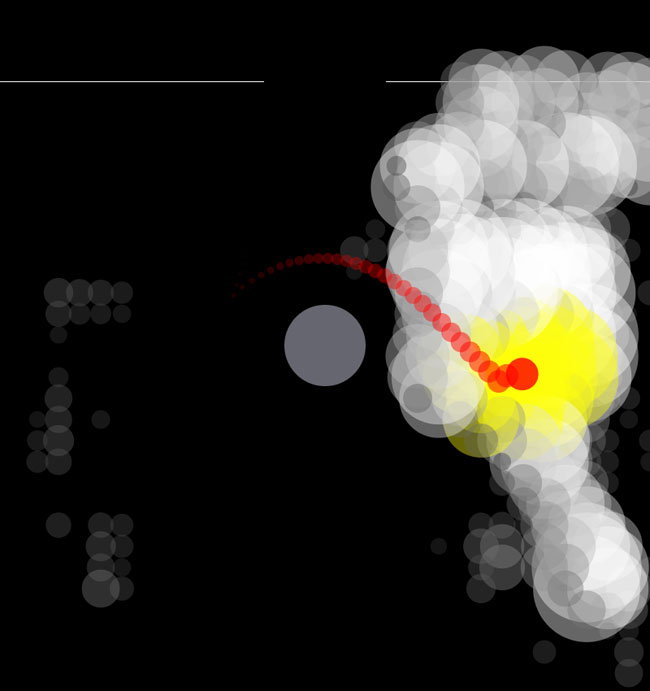
Processing
Main
import processing.video.*; Capture video; PImage prevFrame; float totalMotion; int sGrid = 30; ArrayList data; int counter=0; Ball ball; Obstacle mCircle; float shiftCirclesY=100; import ddf.minim.*; Minim minim; void setup() { smooth(); data= new ArrayList(); video = new Capture(this,800,800,12); prevFrame = createImage(video.width,video.height,RGB); //minim = new Minim(this); size(800, 850); //, OPENGL // create ball objects for (int x = 0; x < sGrid; x++ ) { for (int y = 0; y < sGrid; y++ ) { Obstacle obst = new Obstacle(x*(width/sGrid),y*(width/sGrid)+shiftCirclesY, minim); obst.sGrid = sGrid; data.add(obst); } } mCircle = new Obstacle(mouseX,mouseY,minim); mCircle.mouseC=true; noCursor(); ball = new Ball(shiftCirclesY); } void draw() { background(0); // lines top fill(255); rect(0,shiftCirclesY,width/2-75,1); rect(width/2+75, shiftCirclesY,width/2-75,1); if (video.available()) { prevFrame.copy(video,0,0,video.width,video.height,0,0,video.width,video.height); // Before we read the new frame, we always save the previous frame for comparison! prevFrame.updatePixels(); video.read(); } video.loadPixels(); prevFrame.loadPixels(); pushMatrix(); translate(video.width,0); scale(-1.0, 1.0); counter=0; for (int x = 0; x < sGrid; x++ ) { for (int y = 0; y < sGrid; y++ ) { color average = getAvarageColor(video,x*(video.width/sGrid),y*(video.width/sGrid),video.height/sGrid,video.height/sGrid); color previous = getAvarageColor(prevFrame,x*(video.width/sGrid),y*(video.width/sGrid),video.height/sGrid,video.height/sGrid); float r1m = red(average); float g1m = green(average); float b1m = blue(average); float r2m = red(previous); float g2m = green(previous); float b2m = blue(previous); float diffMotion = dist(r1m,g1m,b1m,r2m,g2m,b2m); int loc = x*y + y; Obstacle tmp = (Obstacle) data.get(counter); tmp.setColors(average); tmp.setBallLoc(ball.location); tmp.draw(); if (tmp.hit==true) { // HIT ball.speedY=-1*abs(tmp.hitLocation.y)*tmp.circleWidth/5; ball.speedX=tmp.hitLocation.x*tmp.circleWidth/5; } counter++; } } // circle center - target mCircle.setColors(color(255)); mCircle.setPos(width/2,height/2); mCircle.setBallLoc(ball.location); mCircle.draw(); if (mCircle.hit==true) { ball.speedY=-1*abs(mCircle.hitLocation.y)*mCircle.circleWidth/5; ball.speedX=mCircle.hitLocation.x*mCircle.circleWidth/5; } ball.draw(); popMatrix(); } color getAvarageColor(PImage _img, int _posX, int _posY, int _w, int _h) { int vR = 0; int vG = 0; int vB = 0; for (int y=_posY; y<_posY+_h; y++) { for (int x=_posX; x<_posX+_w; x++) { vR += red(_img.get(x, y)); vG += green(_img.get(x, y)); vB += blue(_img.get(x, y)); } } return(color(vR/(_w*_h), vG/(_w*_h), vB/(_w*_h))); } void keyPressed() { switch(key) { case 'p': save("screens/points_"+year()+"-"+month()+"-"+day()+"_"+hour()+"-"+minute()+"-"+second()); break; default: break; } // switch }
Class Ball
class Ball { float posX=width/2; float posY=-50; PVector location; PVector velocity; float ballWidth = 40; float speedX; float speedY; float shiftCirclesY; ArrayList whoosh = new ArrayList(); boolean ini=true; Ball(float _s) { location = new PVector(posX, posY); velocity = new PVector(speedX, speedY); whoosh = new ArrayList(); whoosh.add(location); shiftCirclesY=_s; reset(); } void reset() { location.y=0; location.x=width/2; speedX=random(-5,5); speedY=12; ini=true; } void draw() { location.add(velocity); velocity = new PVector(speedX, speedY); if (location.x > width - ballWidth/2) { location.x = width - ballWidth/2; speedX=-1*abs(speedX); } else if (location.x < ballWidth/2) { location.x = ballWidth/2; speedX=1*abs(speedX); } if (ini==false && location.y < ballWidth/2+shiftCirclesY) { speedY=1*abs(speedY); location.y=ballWidth/2+shiftCirclesY; } if (location.y>ballWidth/2+shiftCirclesY) ini=false; ellipseMode(CENTER); fill(255,0,0,204); ellipse(location.x, location.y, ballWidth, ballWidth); posY+=10; // reset when at bottom if (location.y>height) { reset(); } if (speedY<12) speedY++; for(int w=0; w<whoosh.size();w++) { PVector tmp = (PVector) whoosh.get(w); fill(255,0,0,w*4); ellipse(tmp.x,tmp.y,w*0.8,w*0.8); } PVector wtp = new PVector(location.x,location.y); if (whoosh.size()>36) whoosh.remove(0); whoosh.add(wtp); } }
Class Obstacle
class Obstacle { int sGrid = 50; float totalMotion; color average; color previous; float posX; float posY; float targetLength=40; PVector ballLocation; PVector circleLocation; PVector hitLocation; boolean hit=false; float diffMotion; boolean mouseC=false; float circleWidth; AudioSample hitSound; int soundDelay=0; Minim minim; Obstacle(float _x, float _y, Minim _m) { posX = _x; posY = _y; ballLocation = new PVector(0,0); hitLocation = new PVector(0,0); circleLocation = new PVector(posX,posY); //minim = _m; //this.hitSound = minim.loadSample("hit.aiff", 2048); } void setPos(float _x, float _y) { posX = _x; posY = _y; circleLocation = new PVector(posX,posY); } void setColors(color _c) { average = _c; } void setBallLoc(PVector _b) { ballLocation = _b; } float draw() { hit=false; float r1m = red(average); float g1m = green(average); float b1m = blue(average); float r2m = red(previous); float g2m = green(previous); float b2m = blue(previous); diffMotion = dist(r1m,g1m,b1m,r2m,g2m,b2m); diffMotion = map(diffMotion,0,50,0,50); totalMotion += diffMotion; if (targetLength<diffMotion) targetLength=diffMotion; circleWidth = map(targetLength,0,50,0,20); noStroke(); if (mouseC==true) { diffMotion=20; targetLength=152; circleWidth = 100; } float ballDistance = dist(ballLocation.x,ballLocation.y,0,posX,posY,0); ballDistance-=20; fill(targetLength,targetLength,targetLength,102); if (mouseC==true) fill(102,102,112); PVector v3 = ballLocation.cross(circleLocation); if (targetLength>51 && (ballDistance<circleWidth/2)) { hitLocation = PVector.sub(circleLocation,ballLocation); hitLocation.normalize(); hitLocation.x*=-1; fill(255,255,10,102); hit=true; } soundDelay++; if (mouseC==true) { //println(hitLocation); } if (circleWidth<20) circleWidth=0; ellipseMode(CENTER); ellipse(posX, posY, circleWidth, circleWidth); targetLength-=10; previous = average; return ballDistance; } }