März 2, 2012
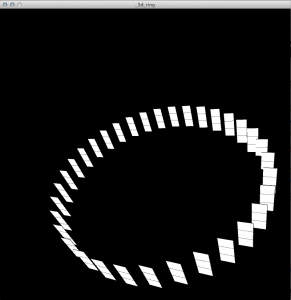
import processing.opengl.*; import peasy.*; PeasyCam cam; int imgSize = 100; int imgCount = 100; int imgRows = 3; int imgPerRow; ArrayList photos; int worldWidth; int worldHeight; float worldX; float worldY; float worldZ; float focusX; float focusY; float destZ = 100; float destY; float destX; float worldRotateX; float worldRotateY; float worldRotateDestX; float worldRotateDestY; void setup() { size(800, 800, OPENGL); cam = new PeasyCam(this, 100); cam.setMinimumDistance(50); cam.setMaximumDistance(5000); smooth(); photos = new ArrayList(); int yPos = 0; imgPerRow = ceil(float(imgCount)/imgRows); float degPerImg = TWO_PI / imgPerRow ; for (int i = 0; i < imgCount;i++) { if (i%imgPerRow == 0) { yPos++; } photos.add(new Photo(cos((i%imgPerRow) * degPerImg) * 1000, yPos, sin((i%imgPerRow) * degPerImg) * 1000, i+1, imgSize)); } worldWidth = imgPerRow*imgSize; worldHeight = imgRows*imgSize; worldZ = 100; addMouseWheelListener(new java.awt.event.MouseWheelListener() { public void mouseWheelMoved(java.awt.event.MouseWheelEvent evt) { mouseWheel(evt.getWheelRotation()); } } ); } void draw() { pushMatrix(); background(0); /* if (worldZ < 3000) { worldRotateDestX = ((PI/2)*mouseY/height-TWO_PI); worldRotateDestY = ((PI/2)*mouseX/width-TWO_PI); destY = (height-worldHeight)/2-60; destY += -(mouseY-height/2)/2; } else { worldRotateDestX = 0; worldRotateDestY = 0; destY = -(mouseY-height/2); } worldY += (destY-worldY)/max((worldZ-100), 25); worldZ += (destZ-worldZ)/50; worldX -= (mouseX-width/2)/max((worldZ-50), 25); worldX = min(max(worldX, -worldWidth+width/2), width/2); worldY = min(max(worldY, -worldHeight+height/2), height/2); worldRotateX += (worldRotateDestX-worldRotateX)/15; worldRotateY += (worldRotateDestY-worldRotateY)/15; translate(width/2, height/2, 0); rotateX(worldRotateX); rotateY(-worldRotateY); scale(worldZ/100); translate(-width/2, -height/2, -0); */ for (int i = 0; i< photos.size();i++) { Photo p = (Photo)photos.get(i); p.drawImage(g); } popMatrix(); } void mouseWheel(int delta) { destZ -= delta*30; if (destZ < 25) { destZ = 25; } } import processing.opengl.*; class Photo { PImage img; float x; float y; float z; float smallWidth; float smallHeight; float currentWidth; float currentHeight; float maxSize; int id; boolean loading; public Photo(float nx, float ny, float nz, int _id,int s) { id = _id; loading = true; x = nx; y = ny; z = nz; maxSize = s; currentWidth = s; currentHeight = s; } public void drawImage(PGraphics g) { g.fill(255); g.beginShape(); g.vertex(x +(maxSize-currentWidth)/2, y*maxSize+(maxSize-currentHeight)/2,z); g.vertex(x+currentWidth+(maxSize-currentWidth)/2, y*maxSize+(maxSize-currentHeight)/2,z); g.vertex(x+currentWidth+(maxSize-currentWidth)/2, y*maxSize+currentHeight+(maxSize-currentHeight)/2,z); g.vertex(x+(maxSize-currentWidth)/2, y*maxSize+currentHeight+(maxSize-currentHeight)/2,z); g.endShape(); } }