Lesson 2.1 – Simple Reactor
Simple Reactor
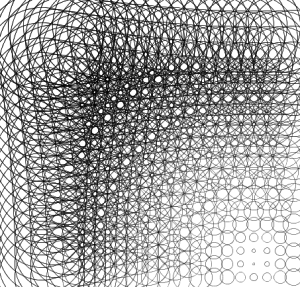
//Reactor //“Make an object respond to the proximity of another object.” PShape basicShape; int arrayWidth = 20; int arrayHeight = 20; float shapeWidth = 20; float shapeHeight = 20; float reactorScaler = .02; int screenWidth = 700; int screenHeight = 700; int xOffset = floor(screenWidth/2-(shapeWidth*arrayWidth/2)); int yOffset = floor(screenHeight/2-(arrayHeight*shapeHeight/2)); PVector reactorPosition; void setup() { reactorPosition = new PVector(0, 0); size(screenWidth,screenHeight); background(255); smooth(); basicShape = loadShape("data/circle.svg"); }; void draw() { background(255); pushMatrix(); //saves current position of the coordinate system translate(xOffset,yOffset);//translate array to center for(int i = 0; i<arrayWidth; i++ ) { for(int j = 0; j<arrayHeight; j++ ) { shapeMode(CENTER); PVector myPos = new PVector(i*shapeWidth, j*shapeHeight); float reactorDistance = dist(reactorPosition.x, reactorPosition.y, myPos.x, myPos.y); float scaler = reactorDistance*reactorScaler; fill(0); shape(basicShape, myPos.x, myPos.y, shapeWidth*scaler, shapeHeight*scaler); stroke(255,0,0); //line(posX, posY, reactorPosition.x, reactorPosition.y); //show line to reactor position }; }; popMatrix(); }; int mouse_X() { return (mouseX - xOffset); //correct positions matrix translations } int mouse_Y() { return (mouseY - xOffset); //correct positions matrix translations } void mouseMoved(){ reactorPosition.x = mouse_X(); reactorPosition.y = mouse_Y(); };
Exercise: Create two new examples by modifying the code with different reactor shapes that respond not only with size change. Try rotation, colour change or deformations.