Lesson 3.3 – (extra) Sine function
Sin function
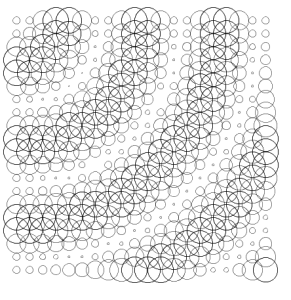
This example uses a similar approach to the last example, with a timer at the the reactor being offset by the distance to each node. What other wave functions could be used to generate patterns in this way, and how could it be made more responsive?
int gridWidth = 20; int gridHeight = 20; float shapeWidth = 20; float shapeHeight = 20; int duration = 100; int duration2 = 50; int scaleTimer = 0; int screenWidth = 800; int screenHeight = 700; int xOffset = floor(screenWidth/2-(shapeWidth*gridWidth/2)); int yOffset = floor(screenHeight/2-(shapeHeight*gridHeight/2)); PVector reactorPosition; ArrayList<graphicObject> graphicList = new ArrayList<graphicObject>(); void setup() { reactorPosition = new PVector(0, 0); size(screenWidth,screenHeight); background(255); smooth(2); for(int i = 0; i<gridWidth; i++ ) { for(int j = 0; j<gridHeight; j++ ) { graphicList.add(new graphicObject(i*shapeWidth, j*shapeHeight)); } } }; void draw() { background(255); scaleTimer++; pushMatrix(); //saves current position of the coordinate system translate(xOffset,yOffset);//translate grid to center for(int i = 0; i<graphicList.size(); i++ ) { graphicList.get(i).draw(reactorPosition); } popMatrix(); }; int mouse_X() { return (mouseX - xOffset); //correct positions matrix translations } int mouse_Y() { return (mouseY - xOffset); //correct positions matrix translations } void mouseClicked(){ scaleTimer = 0; reactorPosition.x = mouse_X(); reactorPosition.y = mouse_Y(); }; class graphicObject { PVector _loc; float _scale = 1.0; graphicObject(float x, float y) { _loc = new PVector(x, y); } void draw(PVector reactor) { float reactorDistance = 0; int myStartTime = 0; float n = 0; reactorDistance = dist(reactor.x, reactor.y, _loc.x, _loc.y); myStartTime = int(scaleTimer-reactorDistance); n = radians(myStartTime)*1.5; _scale = sin(n)*2; pushMatrix(); translate(_loc.x,_loc.y); scale(_scale); noFill(); strokeWeight(0.2); ellipse(0, 0, shapeWidth, shapeHeight); popMatrix(); } }