17. November 2011
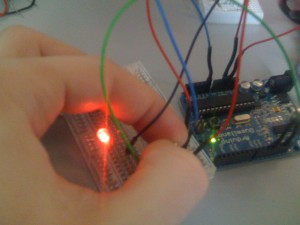
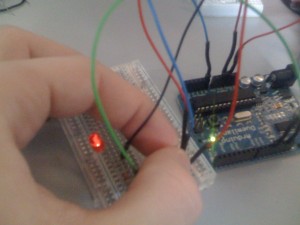

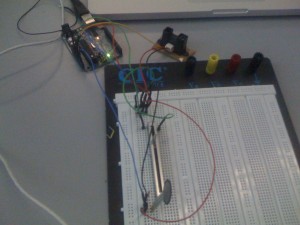
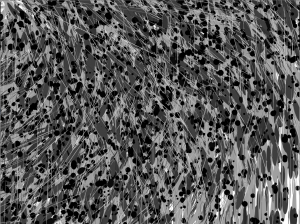
#define POTI_PIN 0 #define POTI_PIN_2 2 #define POTI_PIN_3 3 int potiValue = 0; int potiValue2 = 0; int potiValue3 = 0; void setup(){ Serial.begin(9600); } void loop(){ potiValue = analogRead(POTI_PIN); potiValue2 = analogRead(POTI_PIN_2); potiValue3 = analogRead(POTI_PIN_3); Serial.print(potiValue); // Print the Value Serial.print(','); // Print Delimiter Serial.print(potiValue2); // Print the Value Serial.print(','); // Print Delimiter Serial.print(potiValue3); // Print the Value Serial.print(','); // Print Delimiter Serial.println(); // Print Line Feed }Processing:
import processing.serial.*; // import the serial library Serial myPort; // make an instance of the serial library int potiValue = 0; // Variable to store the incomming value int potiValue2 = 0; int potiValue3 = 0; void setup() { size(1024,768); // Size of the display canvas println(Serial.list()); // Print all aviable Serial ports myPort = new Serial (this, Serial.list()[0], 9600); smooth(); } void draw() { float sensor = map(potiValue, 0, 500, 0, 20); float gleiter = map(potiValue2, 0, 500, 0, 255); float druck = map(potiValue3, 0, 1023, 0, 100); if (druck <= 30 ) { druck= 0; } pushMatrix(); translate(0, 0); for(int x = 1; x <= druck; x++) { if(druck >= 0){ pushMatrix(); translate(random(0, width),random(0,height)); ellipse(0,50,sensor,gleiter+sensor); fill(gleiter); noStroke(); rotate(radians(26*sensor)); popMatrix(); rotate(2); } } popMatrix(); println(druck); println(gleiter); println(potiValue3); } void serialEvent (Serial myPort) { if(myPort.available() > 0) { String completeString = myPort.readStringUntil(10); if(completeString != null) { trim(completeString); String seperateValues[] = split(completeString, ","); potiValue = int(seperateValues[0]); potiValue2 = int(seperateValues[1]); potiValue3 = int(seperateValues[2]); } } }