23. November 2011
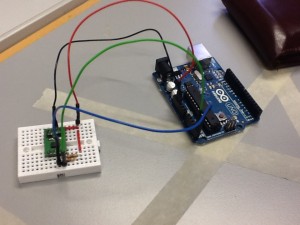
// Includes #include <Wire.h> #include <MMA_7455.h> // Objeczs MMA_7455 sensor = MMA_7455(); // Variables char xNew, yNew, zNew; char xOld, yOld, zOld; // Setup void setup() { // Begin Serial Serial.begin(9600); // Set Sensitivity sensor.initSensitivity(2); // Wait delay(200); // Read Raw Orientation char x = sensor.readAxis('x'); char y = sensor.readAxis('y'); char z = sensor.readAxis('z'); // Calibrate sensor.calibrateOffset(0-x,0-y,64-z); } void loop() { xNew = sensor.readAxis('x'); yNew = sensor.readAxis('y'); zNew = sensor.readAxis('z'); xNew = (0.5*xOld) + (0.5*xNew); yNew = (0.5*yOld) + (0.5*yNew); zNew = (0.5*zOld) + (0.5*zNew); xOld = xNew; yOld = yNew; zOld = zNew; printOrientation(xNew,yNew,zNew); } // Print Orientation to Serial void printOrientation(char x, char y, char z) { Serial.print(x,DEC); Serial.print("\t"); Serial.print(y,DEC); Serial.print("\t"); Serial.print(z,DEC); Serial.print("\t"); Serial.println(); }Processing:
// Includes import processing.serial.*; // Objects Serial serial; // Variables int rawX, rawY, rawZ; int accX, accY, accZ; String lastMode = ""; // Setup Routine void setup() { background(0); size(500,500,P3D); frameRate(30); println(serial.list()); serial = new Serial(this,serial.list()[0],9600); loop(); } // Draw Loop void draw() { // Set Background background(0); // Create String String str = accX+""+accY+""+accZ; // Check if(!lastMode.equals(str)) { // Conditions if(str.equals("002")) { println("Normal"); } else if(str.equals("200")) { println("Links gekippt"); } else if(str.equals("100")) { println("Rechts gekippt"); } else if(str.equals("010")) { println("Hinten gekippt"); } else if(str.equals("020")) { println("Vorne gekippt"); } else if(str.equals("001")) { println("Nach Unten"); } // Save lastMode = str; } } // Serial Data Event void serialEvent(Serial data) { // Check for Data if (data.available() > 0) { // Get whole String String completeString = data.readStringUntil(10); // Check String if (completeString != null) { // Remove whitespace trim(completeString); // Split String seperateValues[] = split(completeString,"\t"); // Set Variables rawX = int(seperateValues[0]); rawY = int(seperateValues[1]); rawZ = int(seperateValues[2]); // Calculate accX = calc(rawX); accY = calc(rawY); accZ = calc(rawZ); } } } // Calculate Value int calc(int in) { if(in >= 40) { return 2; } if(in <= -40) { return 1; } return 0; } // Stop Sketch void stop() { // Close Serial Connection serial.stop(); super.stop(); }