24. November 2011
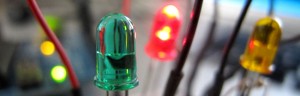
#define BUFFER_SIZE 20 // actual size of the buffer for integer values: (numberOfValsToRead*6)+(numberOfValsToRead-1) #define LED 10 #define LED2 5 #define LED3 3 #define Motor 6 /*SENSOR BEGIN*/ // Read the MMA7455 #include <Wire.h> #include <MMA_7455.h> MMA_7455 mySensor = MMA_7455(); char xVal, yVal, zVal; int xoldVal = 0; int xnewVal = 0; int yoldVal = 0; int ynewVal = 0; int zoldVal = 0; int znewVal = 0; /*SENSOR ENDE*/ char incommingBuffer[BUFFER_SIZE]; // buffer to store incomming values char incomming; // primary buffer to store single incommning bytes int incommingCounter = 0; // counter for counting the positions inside the buffer int firstValue, secondValue, thirdValue; // fourthValue, fifthValue, ... // add more if needed void setup() { Serial.begin(9600); pinMode(LED, OUTPUT); pinMode(Motor, OUTPUT); mySensor.initSensitivity(2); mySensor.calibrateOffset(22, 35, 4); } void readSerial() { while(Serial.available()) { incomming = Serial.read(); // read single incommning bytes if(incomming != '\r') //if no carriage return is received proceed in reading the serial port { incommingBuffer[incommingCounter++] = incomming; // go on the next position in the buffer } else //read until a carriage ('\r') is received { incommingBuffer[incommingCounter] = '\0'; // set the last byte to NULL to sign it for the string operators char *a = strtok(incommingBuffer, ",.;"); // split the string after delimiters into tokens char *b = strtok(NULL, ",.;"); // ... char *c = strtok(NULL, ",.;"); // ... //char *d = strtok(NULL, ",.;"); // add another line if needed firstValue = atoi(a); // convert the strings into integers secondValue = atoi(b); // ... thirdValue = atoi(c); // ... //fourthValue = atoi(d); // add another line if needed incommingCounter = 0; // reset the counter memset(incommingBuffer, 0, BUFFER_SIZE); //overwrite the incommingBuffer } } } void loop() { xVal = mySensor.readAxis('x'); yVal = mySensor.readAxis('y'); zVal = mySensor.readAxis('z'); xnewVal = (0.80*xoldVal)+(0.2*xVal); ynewVal = (0.80*yoldVal)+(0.2*yVal); znewVal = (0.80*zoldVal)+(0.2*zVal); Serial.print(xnewVal, DEC); Serial.print('\t'); Serial.print(ynewVal, DEC); Serial.print('\t'); Serial.print(znewVal, DEC); Serial.print('\t'); Serial.println(); xoldVal = xnewVal; yoldVal = ynewVal; zoldVal = znewVal; readSerial(); // read the values available at the serial port analogWrite(LED, firstValue); analogWrite(Motor, secondValue); /* Serial.print(firstValue); // debugging Serial.print("\t"); Serial.print(secondValue); Serial.print("\t"); Serial.print(thirdValue); Serial.print("\t"); //Serial.print(fourthValue); // add these lines if needed Serial.println(); // send a carriage return for debugging */ }Processing Code
import processing.serial.*; // Import the Processing Serial Library for communicating with arduino Serial myPort; // The used Serial Port int xnewVal, ynewVal, znewVal; // fourthValue, fifthValue, ... // add more if needed int xPosition; float xRotation, yRotation; void setup() { size(500, 500, P3D); background(0); println(Serial.list()); // Prints the list of serial available devices (Arduino should be on top of the list) myPort = new Serial(this, Serial.list()[0], 9600); // Open a new port and connect with Arduino at 9600 baud } void draw() { myPort.write(str(int(map(mouseX,0, 500, 0,255)))); // Write our Variable to Arduino myPort.write(","); // Send a carriage-return to Arduino myPort.write(str(int(map(mouseY,0, 500, 0,255)))); // Write our Variable to Arduino myPort.write(","); // Send a carriage-return to Arduino myPort.write(str(int(map(mouseX,0, 500, 0,255)))); // Write our Variable to Arduino myPort.write(","); // Send a carriage-return to Arduino myPort.write("\r"); //println(int(map(mouseX,0, 500, 0,255))); //delay(200); /*myPort.write(str(0)); // Write our Variable to Arduino myPort.write("\r"); // Send a carriage-return to Arduino delay(200);*/ }