24. November 2011
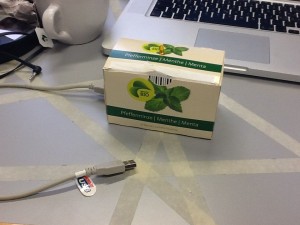
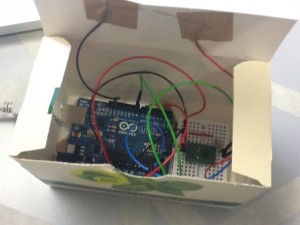
// Constants #define BUFFER_SIZE 20 #define LED 8 #define MOTOR 6 // Includes #include <Wire.h> #include <MMA_7455.h> // Objeczs MMA_7455 sensor = MMA_7455(); // Variables char xNew, yNew, zNew; char xOld, yOld, zOld; // Serial char incommingBuffer[BUFFER_SIZE]; char incomming; int incommingCounter = 0; // Setup void setup() { // Set Led pinMode(LED,OUTPUT); digitalWrite(LED,HIGH); // Set Motor pinMode(MOTOR,OUTPUT); // Begin Serial Serial.begin(9600); // Set Sensitivity sensor.initSensitivity(2); // Wait delay(200); // Read Raw Orientation char x = sensor.readAxis('x'); char y = sensor.readAxis('y'); char z = sensor.readAxis('z'); // Calibrate sensor.calibrateOffset(0-x,0-y,64-z); // Set LED digitalWrite(LED,LOW); } void loop() { readSerial(); xNew = sensor.readAxis('x'); yNew = sensor.readAxis('y'); zNew = sensor.readAxis('z'); xNew = (0.5*xOld) + (0.5*xNew); yNew = (0.5*yOld) + (0.5*yNew); zNew = (0.5*zOld) + (0.5*zNew); xOld = xNew; yOld = yNew; zOld = zNew; printOrientation(xNew,yNew,zNew); } // Print Orientation to Serial void printOrientation(char x, char y, char z) { Serial.print(x,DEC); Serial.print("\t"); Serial.print(y,DEC); Serial.print("\t"); Serial.print(z,DEC); Serial.print("\t"); Serial.println(); } // Read Data from Serial void readSerial() { // Check for Data while(Serial.available()) { // Read Byte incomming = Serial.read(); // Check Byte if(incomming != '\r') { // Add to Buffer incommingBuffer[incommingCounter++] = incomming; } else { // Sign Buffer incommingBuffer[incommingCounter] = '\0'; // Split char *a = strtok(incommingBuffer,",.;"); char *b = strtok(NULL,",.;"); char *c = strtok(NULL,",.;"); int led = atoi(a); int motor = atoi(b); // Set LED digitalWrite(LED,led); // Set Motor analogWrite(MOTOR,motor); // Reset Buffer incommingCounter = 0; memset(incommingBuffer, 0, BUFFER_SIZE); } } }Processing:
// Includes import processing.serial.*; // Objects Serial serial; // Variables int rawX, rawY, rawZ; int accX, accY, accZ; String lastMode = ""; // Setup Routine void setup() { background(0); size(500,500,P3D); frameRate(100); println(serial.list()); serial = new Serial(this,serial.list()[0],9600); loop(); } // Draw Loop void draw() { // Set Background background(0); // Check Serial if(serial.available() > 0) { readSerial(serial); } // Create String String str = accX+""+accY+""+accZ; // Check if(!lastMode.equals(str)) { // Conditions if(str.equals("002")) { println("Normal"); serial.write("1;0;0;\r"); } else if(str.equals("200")) { println("Links gekippt"); serial.write("0;255;0;\r"); } else if(str.equals("100")) { println("Rechts gekippt"); serial.write("0;255;0;\r"); } else if(str.equals("010")) { println("Hinten gekippt"); serial.write("0;255;0;\r"); } else if(str.equals("020")) { println("Vorne gekippt"); serial.write("0;255;0;\r"); } else if(str.equals("001")) { println("Nach Unten"); serial.write("0;255;0;\r"); } // Save lastMode = str; } } // Serial Data Event void readSerial(Serial data) { // Check for Data if (data.available() > 0) { // Get whole String String completeString = data.readStringUntil(10); // Check String if (completeString != null) { // Remove whitespace trim(completeString); // Split String seperateValues[] = split(completeString,"\t"); // Check if(seperateValues.length >= 3) { // Set Variables rawX = int(seperateValues[0]); rawY = int(seperateValues[1]); rawZ = int(seperateValues[2]); // Calculate accX = calc(rawX); accY = calc(rawY); accZ = calc(rawZ); } } } } // Calculate Value int calc(int in) { if(in >= 40) { return 2; } if(in <= -40) { return 1; } return 0; } // Stop Sketch void stop() { // Close Serial Connection serial.stop(); super.stop(); }